Steve Reich’s Clapping Music¶
In this example, we will use some of auxjad
’s classes and functions to
generate the score of Steve Reich’s Clapping Music.
First, we start by importing both abjad
and auxjad
.
>>> import abjad
>>> import auxjad
Let’s now input the basic material that will be used to generate this
composition. The original score is notated using a rhythmic staff, so we can
initialise abjad.Staff
with the property
lilypond_type
set to
"RhythmicStaff"
.
>>> material = abjad.Staff(r"\time 12/8 c8 c c r c c r c r c c r",
... lilypond_type="RhythmicStaff",
... )
>>> abjad.show(material)

Next, we create an instance of auxjad.Phaser
which will be used to
create the phasing process of the initial material. We initialise it with
material
as well as a step_size
of the length of a
quaver.
>>> phaser = auxjad.Phaser(material,
... step_size=abjad.Duration((1, 8)),
... )
Since Reich’s composition phases the material until it is back at its initial
position, we can use the method output_all()
to generate
all thirtee measures of the bottom staff.
>>> notes = phaser.output_all()
>>> phased_staff = abjad.Staff(notes,
... lilypond_type="RhythmicStaff",
... )
>>> abjad.show(phased_staff)
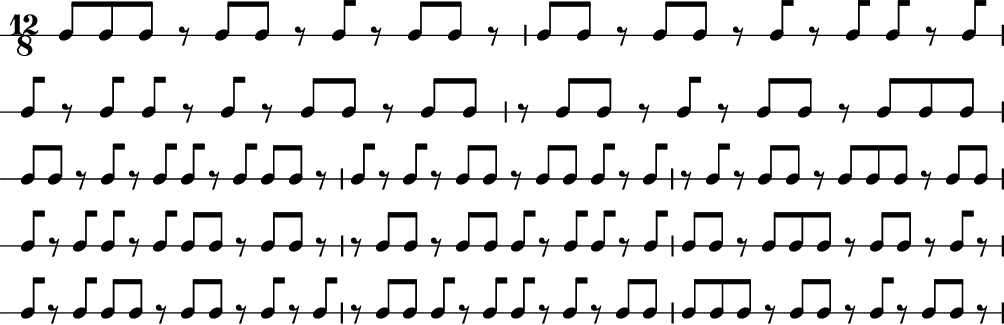
The upper staff of the composition consists of thirteen measures of the
material being repeated. We can thus use the class auxjad.Repeater()
to
generate these repetitions and take care of removing the time signatures of the
repeated measures.
>>> repeater = auxjad.Repeater(material)
>>> notes = repeater(13)
>>> constant_staff = abjad.Staff(notes,
... lilypond_type="RhythmicStaff",
... )
>>> abjad.show(constant_staff)
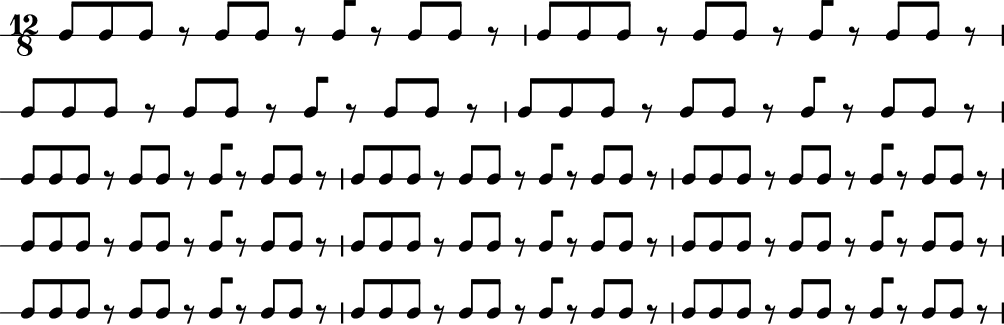
With both staves created, we can now add them to a single score.
>>> score = abjad.Score([constant_staff, phased_staff])
>>> abjad.show(score)
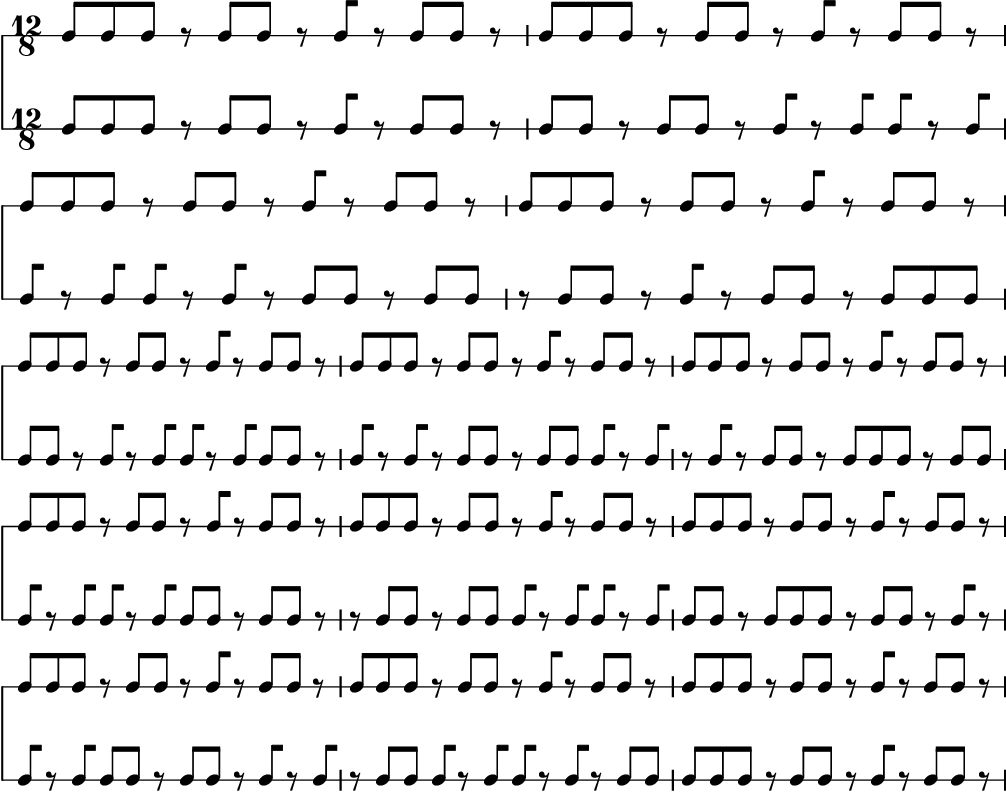
We now group the leaves of the upper staff by measures and add a double repetition bar line to the last leaf of each measure. The very last leaf of the score should have a single end repetition bar line.
>>> measures = abjad.select(constant_staff[:]).group_by_measure()
>>> for measure in measures[:-1]:
... abjad.attach(abjad.BarLine(':..:'), measure[-1])
>>> abjad.attach(abjad.BarLine(':|.'), constant_staff[-1])
>>> abjad.show(score)
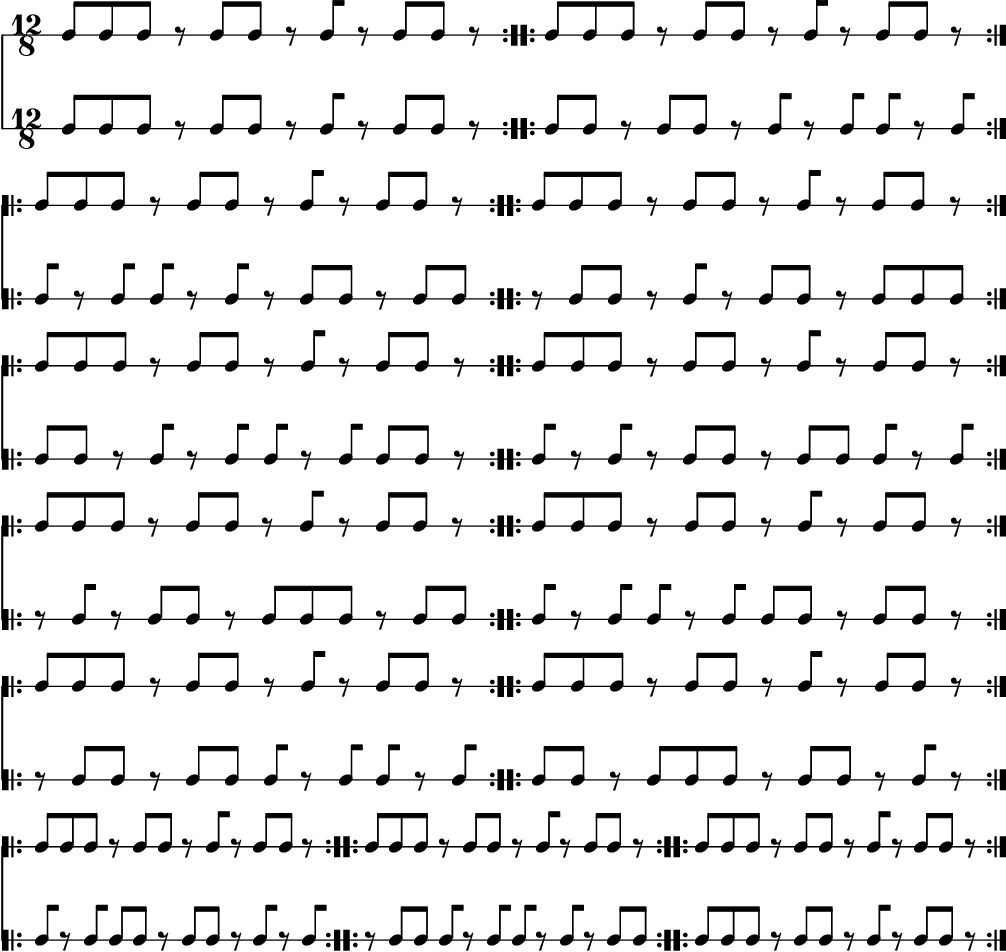