Looping with auxjad.WindowLooper
¶
In this next example, we will use some of auxjad
’s classes to generate
a container of randomly selected material, and then use this material as input
for the looping and shuffling classes.
First, we start by importing both abjad
and auxjad
.
>>> import abjad
>>> import auxjad
Let’s start by deciding what random selectors will be responsible for
generating each parameter of our basic material. Let’s use
auxjad.TenneySelector
for pitches, which is an implementation of
Tenney’s Dissonant Counterpoint Algorithm; at each call, this algorithm
prioritises elements that haven’t been select for the longest time. For the
durations, dynamics, and articulations, the example will use
auxjad.CartographySelector
. Each element input into this type of
selector has a probability of being selected which is dependent on its index.
By default, the probability of consecutive elements decay with a rate of
0.75
. For more information on both of these classes, check auxjad
’s API
page (link in the left panel).
>>> pitch_selector = auxjad.TenneySelector(["c'",
... ("fs'", "g'"),
... "ef''",
... "a''",
... ("b", "bf''"),
... ])
>>> duration_selector = auxjad.CartographySelector([(2, 8),
... (3, 8),
... (5, 8),
... ])
>>> dynamic_selector = auxjad.CartographySelector(['p', 'mp', 'mf', 'f'])
>>> articulation_selector = auxjad.CartographySelector([None, '-', '>'])
Let’s now create eight random notes, each with four parameters randomly selected by the classes above.
>>> pitches = [pitch_selector() for _ in range(8)]
>>> durations = [duration_selector() for _ in range(8)]
>>> dynamics = [dynamic_selector() for _ in range(8)]
>>> articulations = [articulation_selector() for _ in range(8)]
With these list
’s’ of pitches, durations, dynamics, and articulations,
we can now use auxjad.LeafDynMaker
to create the individual abjad
leaves for us. It’s important to note that there is no time signature being
imposed at this point, so LilyPond will fallback to a four by four when
displaying the container below. This is not a problem since this will be used
as the basic material for the looper, which will then automatically take care
of time signatures.
>>> leaf_dyn_maker = auxjad.LeafDynMaker()
>>> notes = leaf_dyn_maker(pitches, durations, dynamics, articulations)
>>> container = abjad.Container(notes)
>>> abjad.show(container)
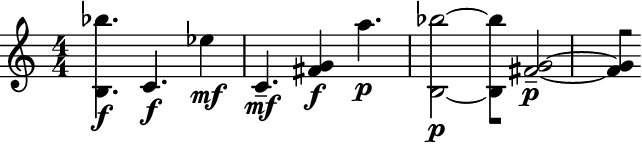
Let’s now use auxjad.WindowLooper
to output loops of windows of the
material. Let’s initiate this class with a window size of a 4/4 measure, and a
step forward of a semiquaver. Setting
after_rest
to the duration (1, 4)
will add
crotchet rests in between consecutive outputs of this looper. Setting
after_rest_in_new_measure
to True
ensure that
these rests (which work as separators of consecutive windows) are in a new
measure by themselves.
>>> looper = auxjad.WindowLooper(container,
... window_size=(4, 4),
... step_size=(1, 16),
... after_rest=(1, 4),
... after_rest_in_new_measure=True,
... )
We can now use the output_n()
to output several
measures of the looping process for us. In this case, let’s output four
measures.
>>> staff = abjad.Staff()
>>> notes = looper.output_n(4)
>>> staff.append(notes)
>>> abjad.show(staff)
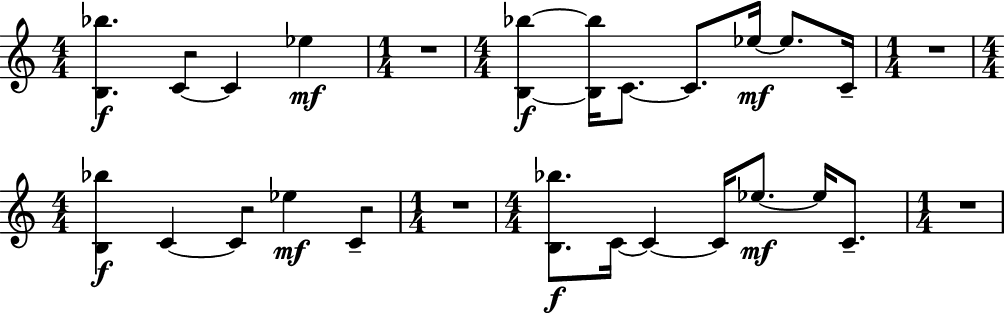
Let’s now change the values of step_size
from a
semiquaver into a crotchet and output four more measures.
>>> looper.step_size = (1, 4)
>>> notes = looper.output_n(4)
>>> staff.append(notes)
>>> abjad.show(staff)
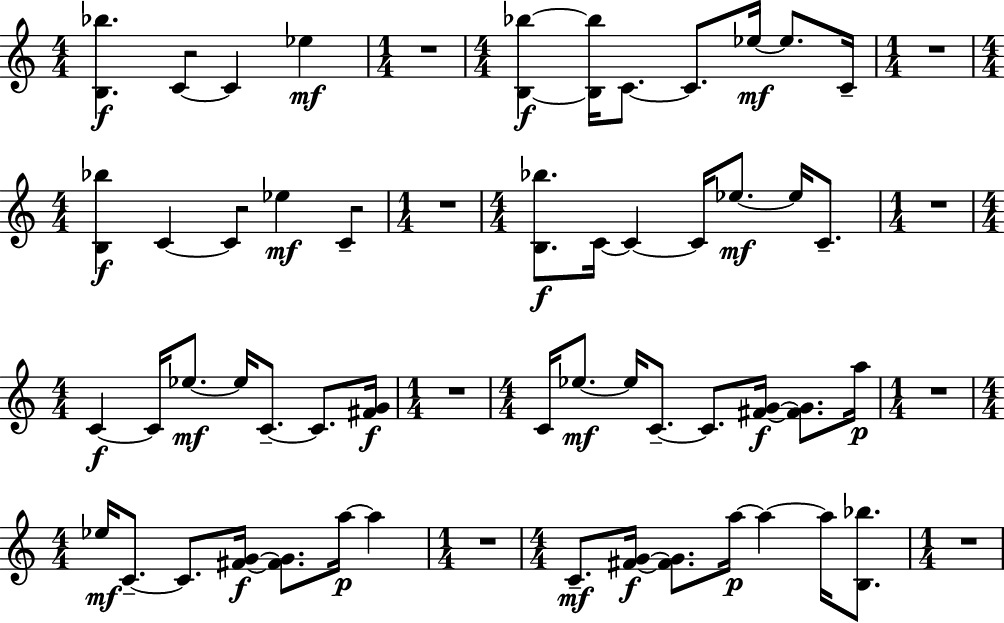
Let’s now change both window_size
and
step_size
as well as remove the after rests, and
then output six more measures.
>>> looper.window_size = (7, 8)
>>> looper.step_size = (1, 16)
>>> looper.after_rest = 0
>>> notes = looper.output_n(6)
>>> staff.append(notes)
>>> abjad.show(staff)
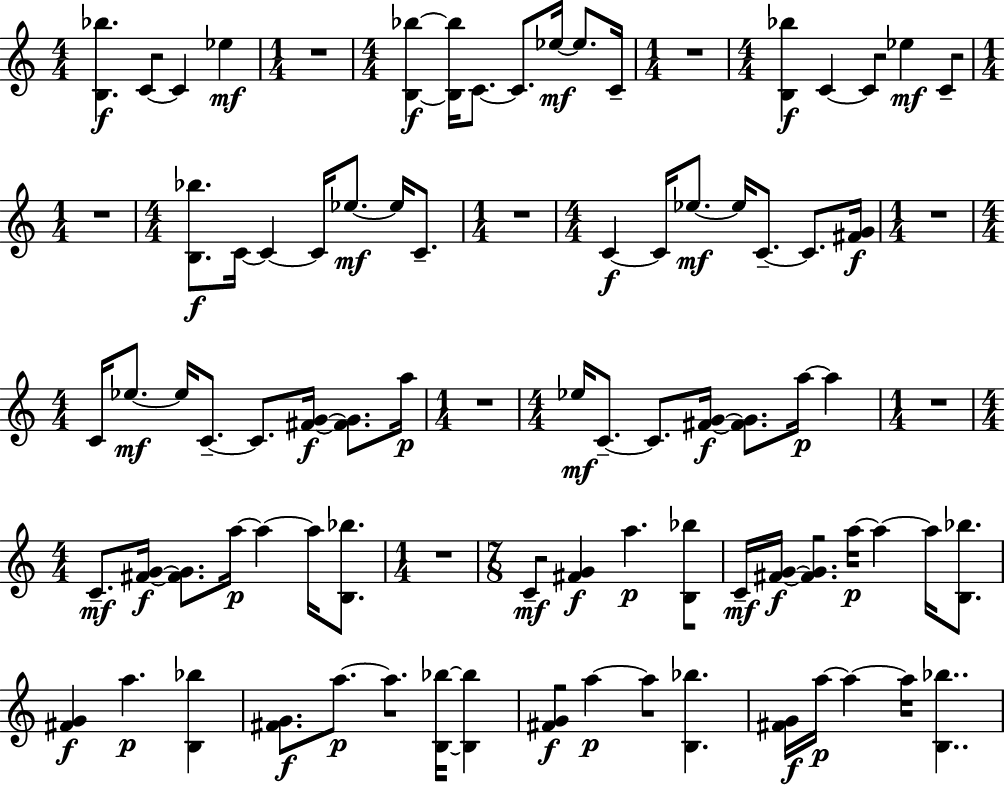
Let’s now add this staff to an abjad.Score
and call the method
add_final_bar_line()
which Auxjad adds to abjad.Score
.
>>> score = abjad.Score([staff])
>>> score.add_final_bar_line()
The final result is shown below.
>>> abjad.show(score)
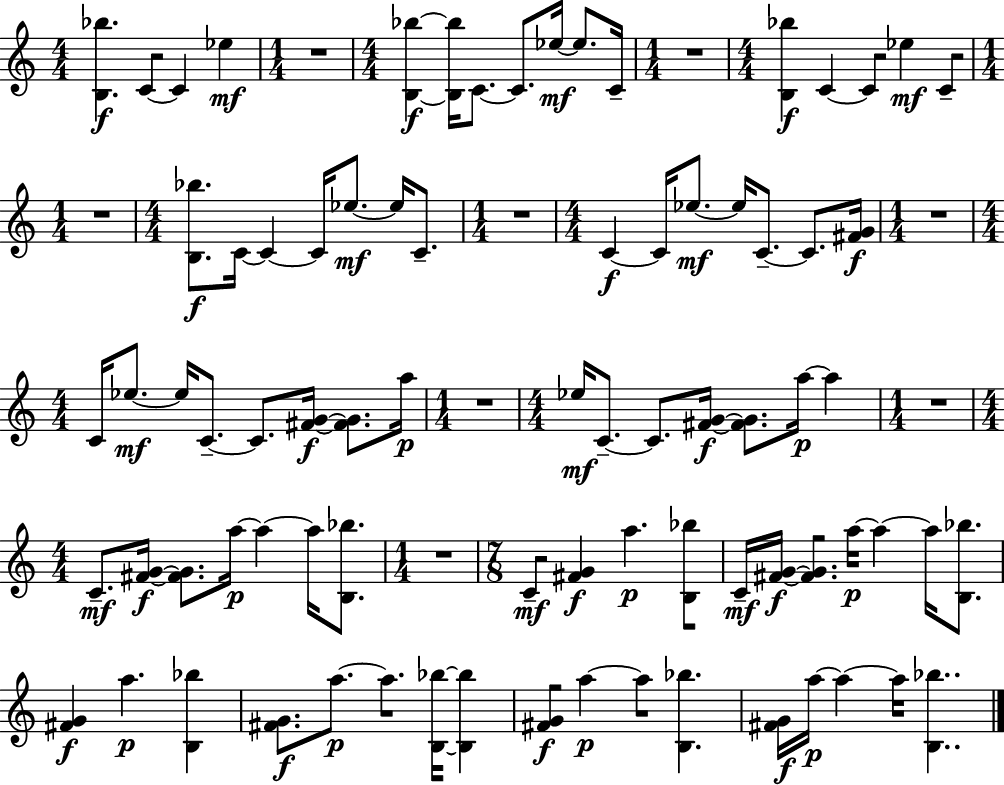