Random repetitions¶
In this example, we will use some of auxjad
’s classes to generate random
repeated measures.
First, we start by importing both abjad
and auxjad
.
>>> import abjad
>>> import auxjad
First, let’s create a container with an arbitrary rhythm. The pitches do not matter at this point since we will randomise them shortly.
>>> container = abjad.Container(
... r"c'4-- c'8.-- c'16( c'8)-. c'8-. c'8-. r8"
... )
>>> abjad.show(container)
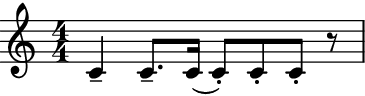
Next, let’s initialise auxjad.PitchRandomiser
with this container as
well as a list
representing pitches. This will be the source for the
random pitches selected by this randomiser.
>>> pitch_list = ["c'", "cs'", "d'", "ef'", "e'"]
>>> randomiser = auxjad.PitchRandomiser(container,
... pitches=pitch_list,
... )
Let’s now output a first group of two measures with random pitches using the
method auxjad.PitchRandomiser.output_n()
.
>>> notes = randomiser.output_n(2)
>>> group_1 = abjad.Staff(notes)
>>> abjad.show(group_1)

Let’s now change the pitch list
using the property
pitches
of the randomiser and create another
group of measures.
>>> randomiser.pitches = ["a", "b", "bf'", "a''", "b''"]
>>> notes = randomiser.output_n(2)
>>> group_2 = abjad.Staff(notes)
>>> abjad.show(group_2)

Up to now, the pitches were being selected with equal weight (i.e. an uniform
distribution). Changing the weights
property to
a list
of int
’s or float
’s allow us to give more weight to
certain pitches. It’s important that this list
has the same length as
the number of pitches in pitches
.
>>> randomiser.weights = [6, 3, 2, 1, 1]
At this point, let’s also change the input container for the randomiser:
>>> container = abjad.Container(
... r"\time 3/4 c'4--( ~ "
... r"\times 4/5 {c'16 c'16-. c'16-. c'16-. c'16-.)} "
... r"r8 c'8->"
... )
>>> abjad.show(container)
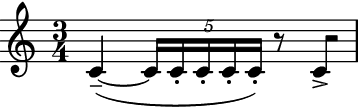
Now, with the new contents of the randomiser, let’s output two more measures as the third and final group of measures.
>>> randomiser.contents = container
>>> notes = randomiser.output_n(2)
>>> group_3 = abjad.Staff(notes)
>>> abjad.show(group_3)
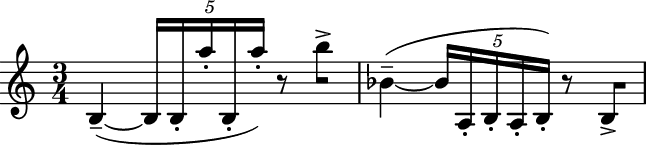
We can now use auxjad.Repeater
to create a staff made out of multiple
repetitions of these three groups. When the repeater type is set to to
'volta'
using the attribute repeat_type
, it will
output measures with repetition bar lines and with a written indication for the
number of repeats. Let’s start with the first group and repeat it 3x.
>>> staff = abjad.Staff()
>>> repeater = auxjad.Repeater(group_1,
... repeat_type='volta',
... )
>>> notes = repeater(3)
>>> staff.append(notes)
>>> abjad.show(staff)

Let’s now do the same with the second group, repeating it 5x and appending it to our staff.
>>> repeater.contents = group_2
>>> notes = repeater(5)
>>> staff.append(notes)
>>> abjad.show(staff)

Finally, let’s do the same with the third group and repeat it 4x. This will be our final score.
>>> repeater.contents = group_3
>>> notes = repeater(4)
>>> staff.append(notes)
>>> abjad.show(staff)
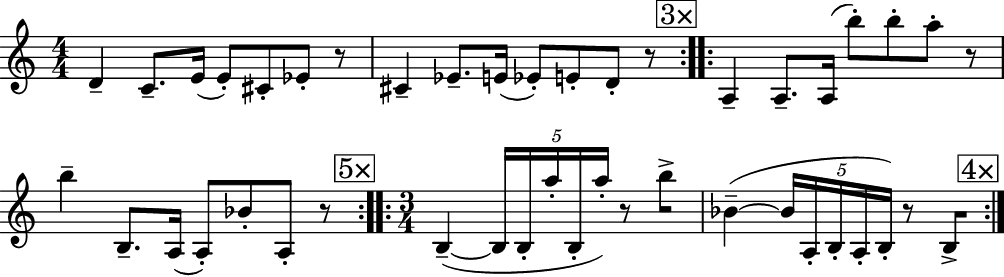